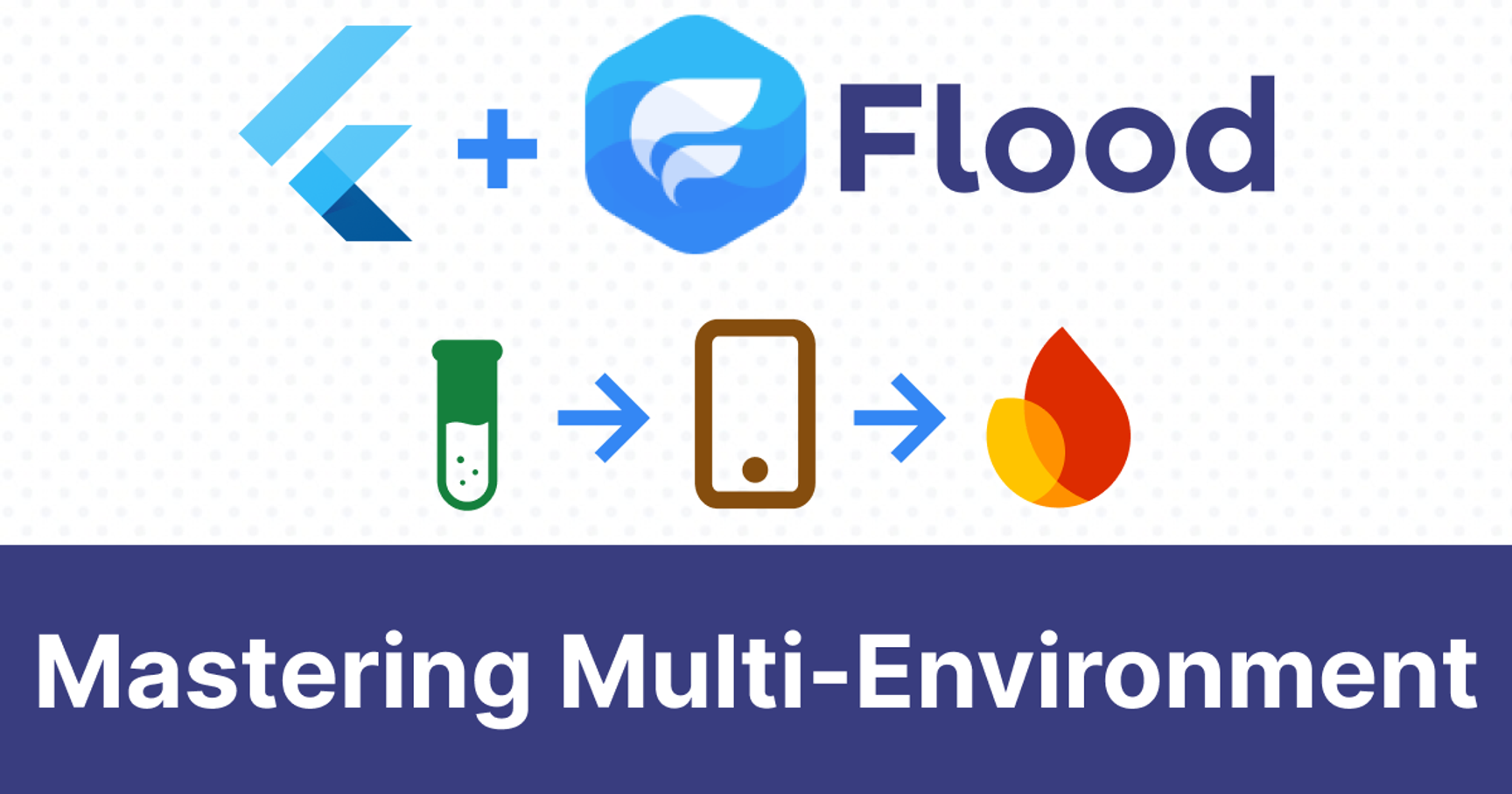
Mastering Multi-Environment
From Local to Production

Jake Boychenko
Published August 9, 2024
Flutter developers often face a significant challenge: managing multiple environments throughout the app development lifecycle. From local testing to production deployment, each stage requires different configurations, data sources, and behavior. This complexity can lead to inconsistencies, bugs, and deployment headaches.
Enter FloodHome, an open-source Flutter toolkit that revolutionizes multi-environment management. In this post, we'll explore how Flood simplifies this process, allowing you to seamlessly transition your app from development to production.
By the end of this article, you'll understand:
- The importance of multi-environment development
- How Flood's Environment Module works
- Best practices for setting up and managing multiple environments
- Techniques for testing across different environments
Understanding Multi-Environment Development
Multi-environment development is the practice of running your app under different configurations to simulate various scenarios it might encounter. It's crucial for ensuring your app behaves correctly in all situations and offers significant benefits in terms of resource management and cost efficiency.
Common environments in Flood include:
- Testing: Simulates all services in-memory. Perfect for unit tests and rapid development cycles. Data resets on app restart.
- Device: Simulates services on the device's file system. Ideal for integration testing and mimicking real-world usage. Data persists between app restarts.
- Staging: Uses online resources (like Firebase) but in a separate instance from production. Great for final testing before release.
- Production: The live environment where real users interact with your app.
Flutter developers often grapple with several challenges when managing multiple environments. These include ensuring consistent app behavior across different settings, handling environment-specific configurations, efficiently transitioning between environments, and maintaining proper data isolation. These hurdles can significantly complicate the development process, potentially leading to inconsistencies, bugs, and deployment issues if not addressed effectively.
Flood's Approach to Environment Management
Flood's Environment Module offers a elegant solution to these challenges. It provides:
- Automatic environment detection and configuration
- Easy switching between environments
- Seamless integration with other Flood modules
For example, the Drop Module (Flood's data layer) automatically adapts its data source based on the current environment. In the testing environment, it uses in-memory storage, while in production, it might use Firebase.
Similarly, the Auth Module can use a mock authentication service in the testing environment and switch to Firebase Auth in production.
A crucial feature of Flood is its ability to simulate repository security rules in the testing and device environments. This means you can verify and test your security rules locally before deploying them to Firebase, catching potential issues early in the development process.
Key benefits of Flood's approach:
- Reduced boilerplate code
- Consistent behavior across environments
- Easy testing and debugging
- Smooth transition from development to production
- Early validation of security rules without risking production data
This comprehensive environment management system ensures that your app behaves consistently and securely across all stages of development, from local testing to production deployment.
Setting Up Your Flutter Project for Multi-Environment Support
Flood promotes a clean and intuitive project structure for managing environments:
1. Create environment-specific configuration files: In your assets
folder, create files like:
config.testing.yaml
config.device.yaml
config.staging.yaml
config.production.yaml
Each file can contain environment-specific values like:
api_url: <https://api.myapp-staging.com>
feature_flags:
new_ui_enabled: true
2. Use config.overrides.yaml
for development: This file overrides environment values while you're developing. It's perfect for setting the environment you want to run in:
environment: testing
This file should be git-ignored to prevent accidental commits of development settings.
3. Flood's FloodCoreComponent
handles the rest: The beauty of Flood is that once you've set up these configuration files, you don't need to do anything else. The FloodCoreComponent automatically reads the correct configuration based on the current environment. In your main.dart
: This single line sets up your entire environment management system!
await corePondContext.register(FloodCoreComponent(
environmentConfig: EnvironmentConfig.static.flutterAssets(),
));
Testing
Flood's Testing Module allows you to customize the testing environment with mock data, ensuring your app behaves correctly in all scenarios.
Here's a simple example:
await appPondContext.register(TestingSetupAppComponent(
onSetup: () async {
// Create a test user
final account = await context.authCoreComponent.signup(
AuthCredentials.email(email: 'test@example.com', password: 'password')
);
// Create some test data
await context.dropCoreComponent.updateEntity(TodoEntity()
..set(Todo()
..nameProperty.set('Buy groceries')
..ownerProperty.set(account.accountId)));
},
));
This setup creates a test user and some initial data every time your app starts in the testing environment, providing a consistent starting point for your tests.
Conclusion
Proper environment management is crucial for developing robust, production-ready Flutter apps. Flood's approach simplifies this complex task, allowing you to focus on building great features instead of wrestling with environment configurations.
By leveraging Flood's Environment Module along with its integration across other modules like Drop and Auth, you can achieve:
- Consistent behavior across all stages of development
- Easier testing and debugging
- Smoother transitions from development to production
We encourage you to implement these practices in your Flutter projects. The time you invest in setting up proper environment management will pay dividends in reduced bugs, faster development cycles, and more confident deployments.
To dive deeper into Flood's environment management capabilities, check out the Environment Module documentation. And if you're new to Flood, our Install & Run Your First Flood App guide is the perfect place to start your journey to more efficient Flutter development.
Happy coding, and may your deployments always be smooth!